Supplier Connections - Developer Resources
>>>Add Supplier Connection
STEP 1
To add a new supplier connection - On Dashboard - Go to Settings -> Configuration -> Supplier Connections.
Suppliers column must be present in the configuration table.
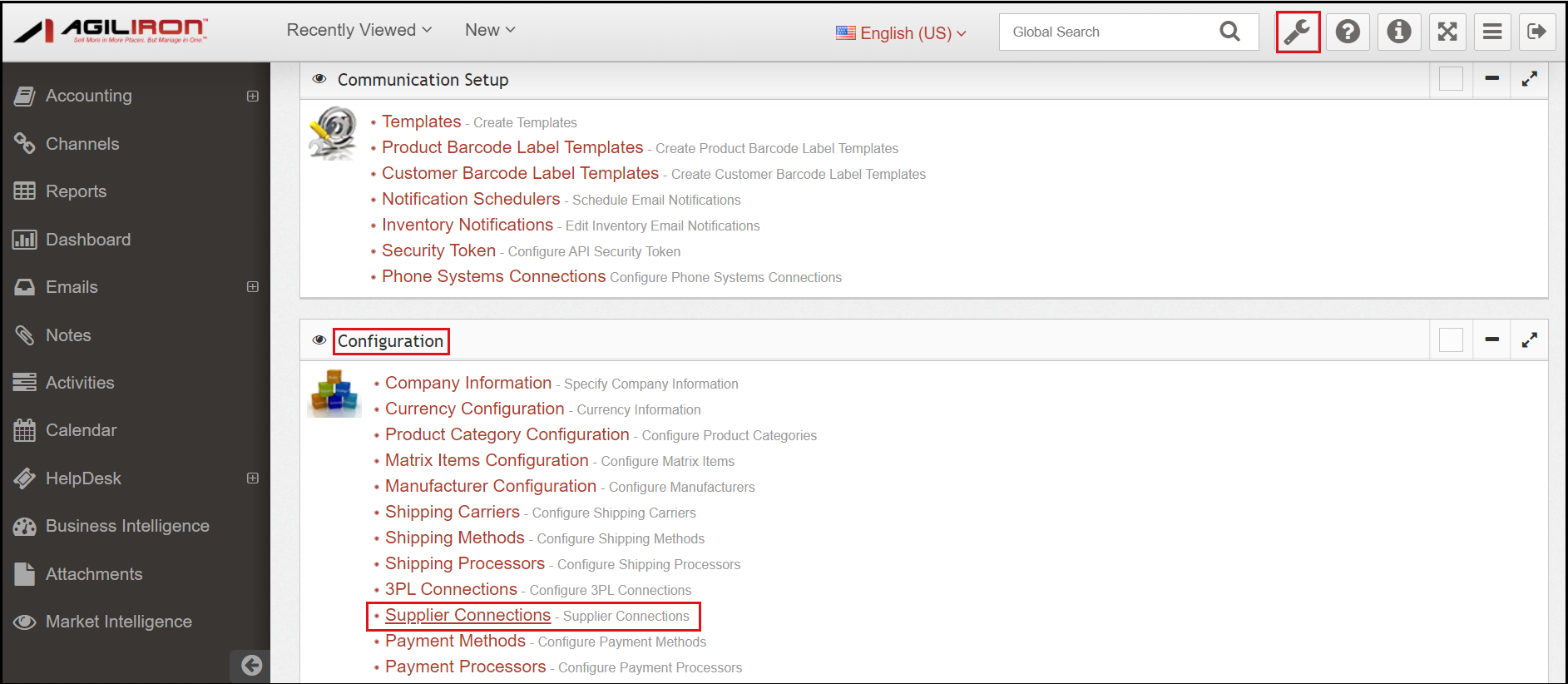
STEP 2
- Supplier Connections page will open.
- To add a new Supplier Connection, click on Add Supplier Connection button.
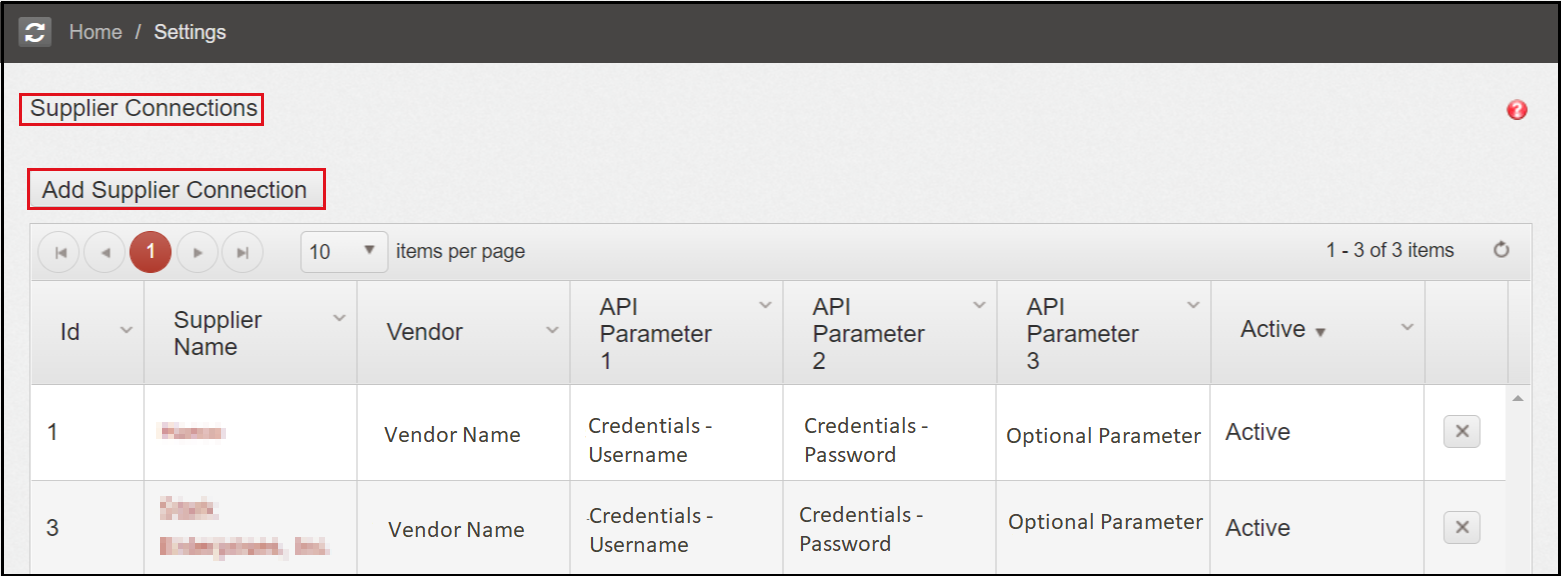
STEP 3
- This will bring up a new form page where you can add a new supplier connection.
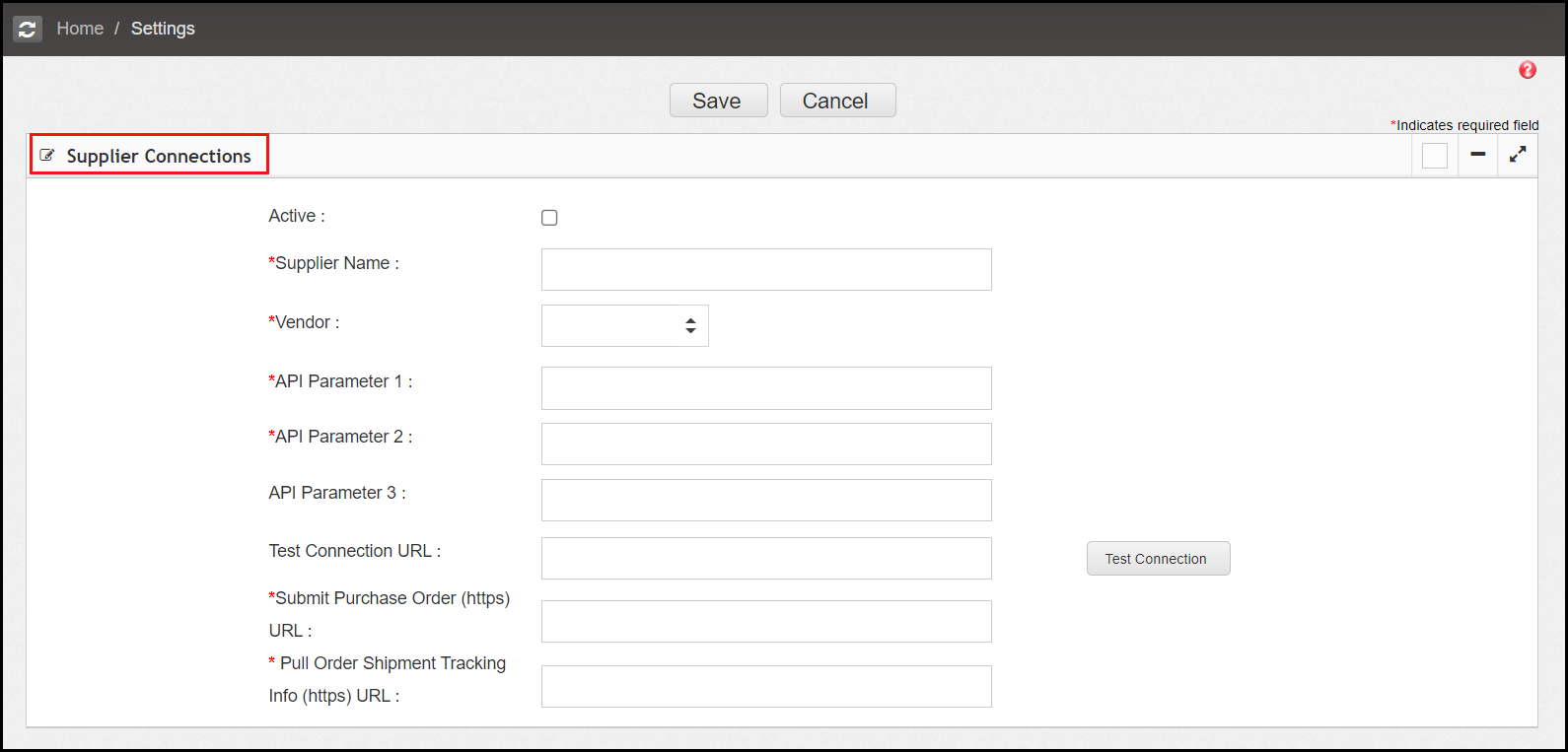
-
Here, fill in the configuration information of the supplier and add its API credentials and then click on Save.
-
Before saving, test whether the API credentials entered, are valid or not by clicking on the Test Connection button.
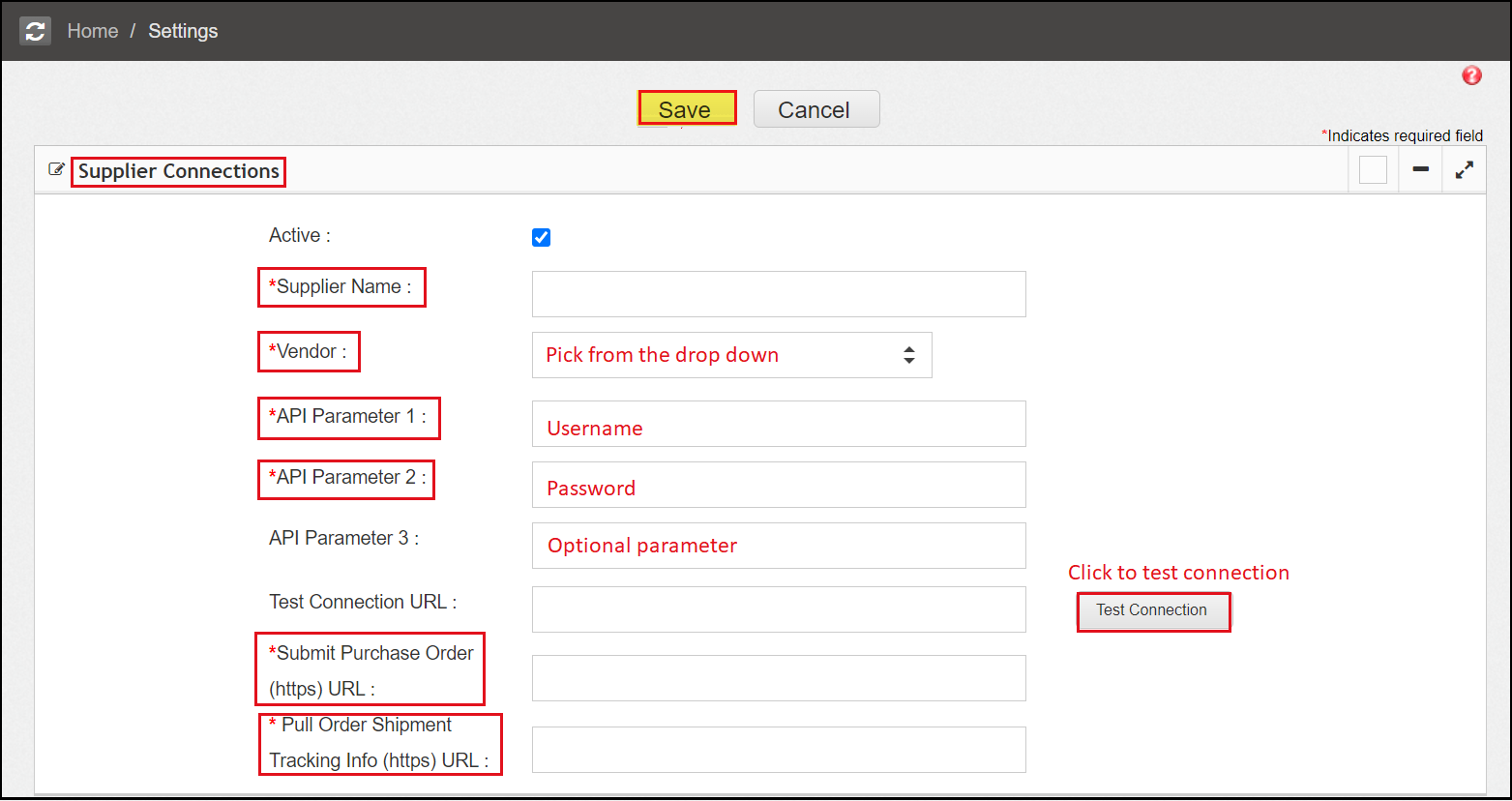
If the API credentials passed are valid, a pop-up message will appear saying, 'Test Connection Successful'.
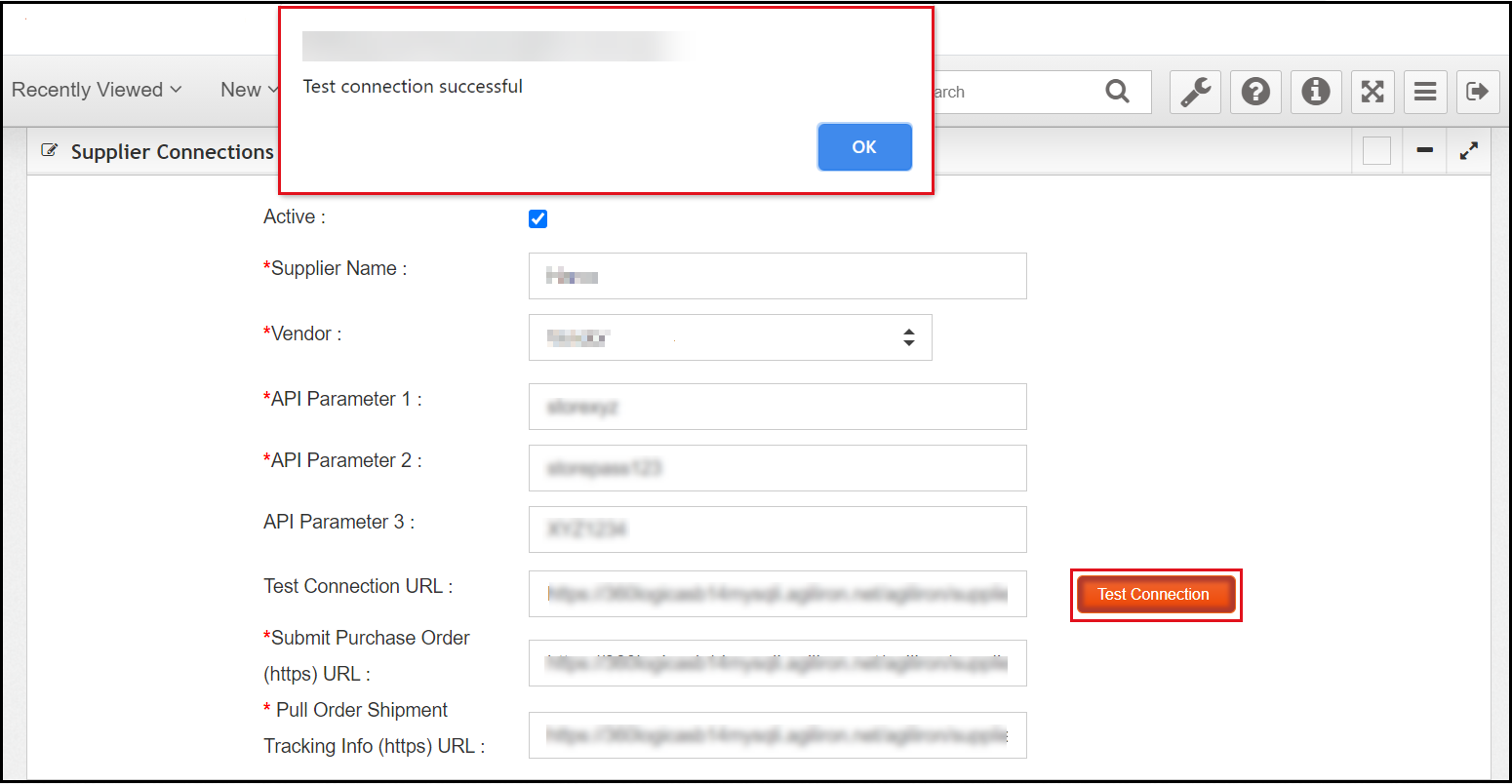
If the credentials are invalid, a pop-up message will appear saying, 'Test Connection Failed'.
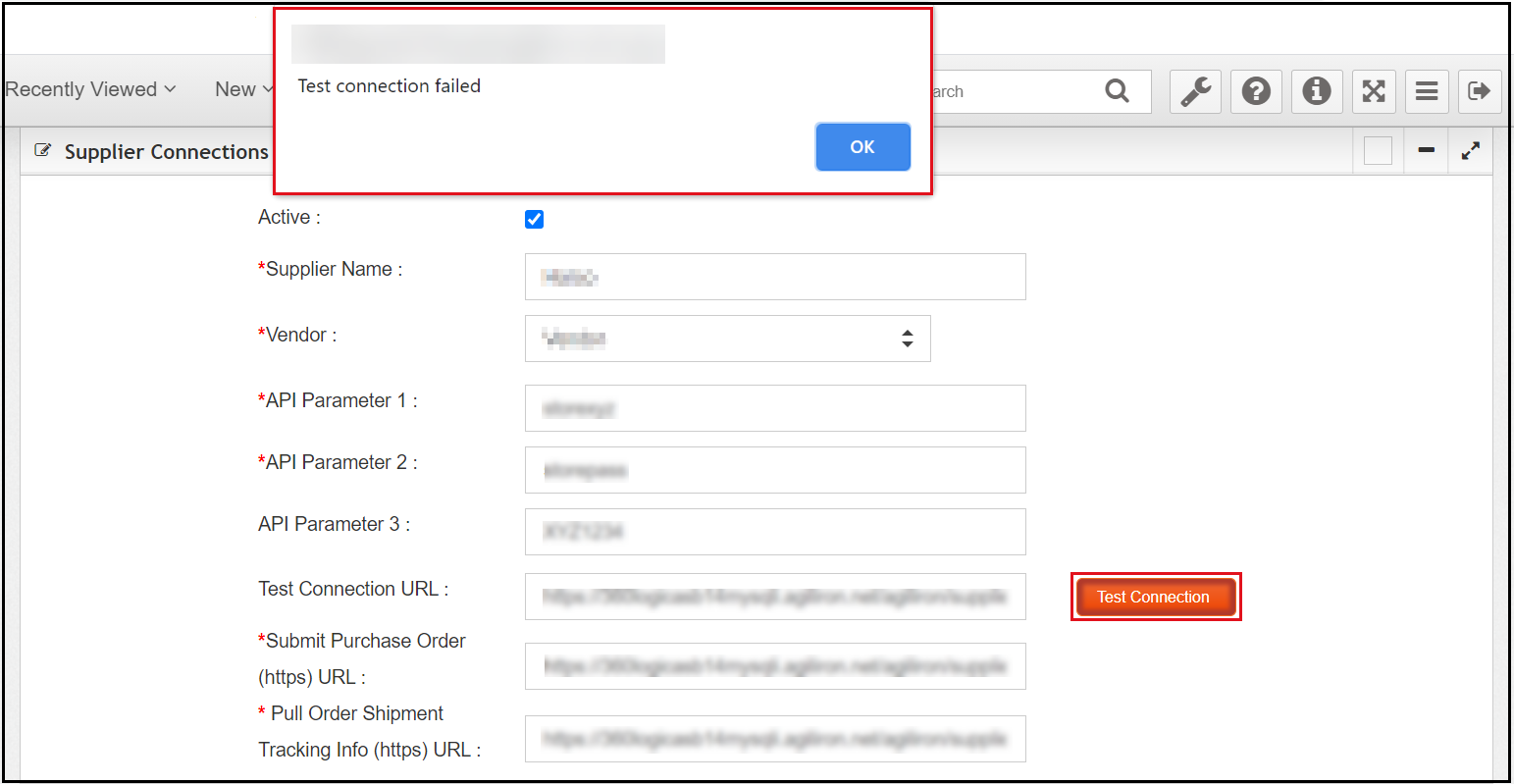
While saving any supplier in the supplier configuration, the following information gets stored:
- Supplier Name - The name of the supplier
- Vendor - Vendor which relates to the supplier. The above supplier configuration will be used for the selected vendor
- API Parameter1 - API credentials (username)
- API Parameter2 - API credentials (password)
- API Parameter3 - Optional parameter
- Test Connection URL - This URL will have a code that will check whether the passed API Parameters are valid or not
- Submit Purchase Order URL - This URL will have a code to create an order by using the class files created in the module. This will be the endpoint
- Pull Order Shipment Tracking Information URL - The URL will have a code to get the shipment information and update the Agiliron order using the class files
- To edit the supplier connection, click on the supplier name from the list.
- To delete the supplier connection, click on the 'x' sign.
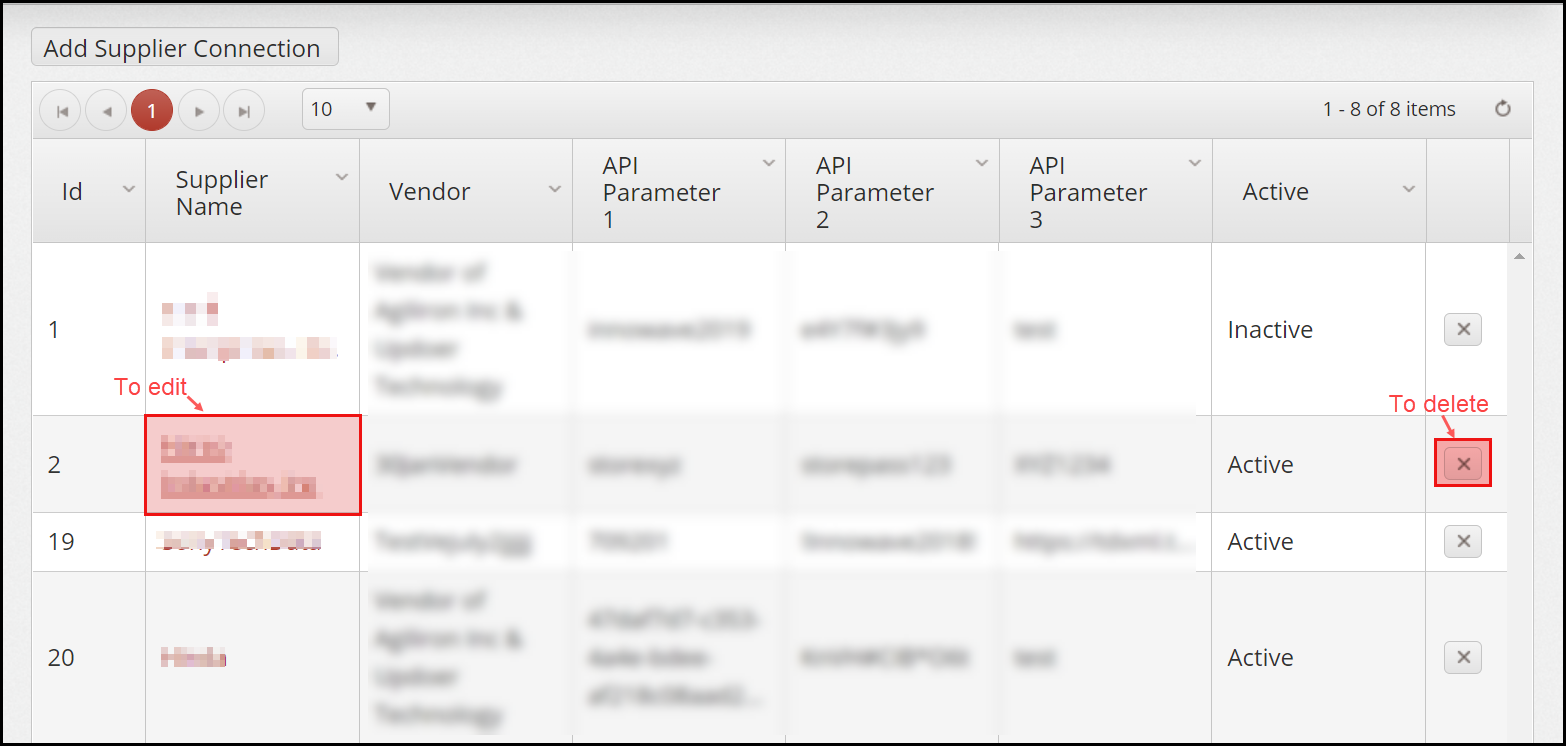
STEP 4
After adding the supplier connection, a supplier folder needs to be created under the supplierconnectors folder as shown below:
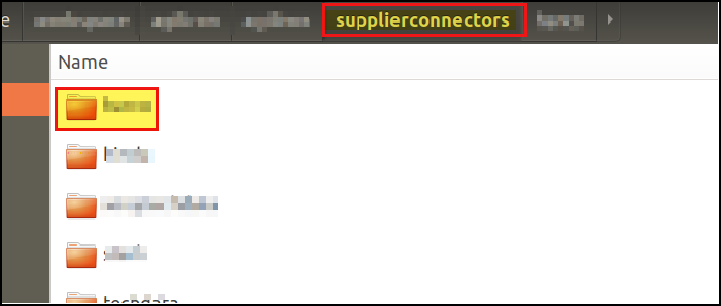
STEP 5
- Need to create class file supplier as below:
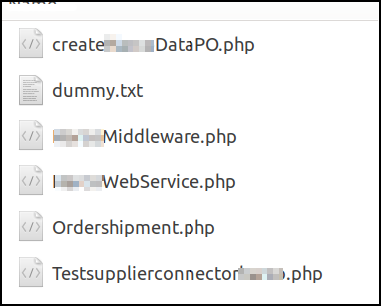
>>>Sending Purchase Order to the Supplier
For sending the purchase order, the order needs to be associated with the vendor that you have configured. While creating a supplier connection we have added a vendor to which it relates to.
Now to send an order to that supplier we need to open purchase order.
In Purchase Order, there will be two buttons that will use these files in the coding flow.

a) Transmit to (Supplier name)
By clicking on the Transmit to (Supplier name) button, the purchase order will be sent to the associated supplier.
The order will be created/accepted only if the sent order has the matching products/line items available at the supplier's end or else it will throw an error. While validating it checks the vendor part number.
Below are the related files:
1. createVendorDataPO.php
- In this file we read request parameters and based on these parameters we simplify the data and convert it to proper array structure.
- If user id and password are not blank in the request parameter then we call the createVendorOrder function for getting the response in VendorMiddleware.php.
2. VendorMiddleware.php
It basically has three main functions as stated below:
createVendorOrder -
- In this we call the function from webservice createVendorOrder
- Based on this function response, we check the generated XML request and post this request by curl call and return the response as per curl response.
checkAuthentication -
- In this function we call getcheckAuthentication which is defined in VendorWebservice.php and post a get method for checking whether the connection is working or not and based on curl response we return the response.
getPOStatus -
- In this function we call the getVendorOrderstatus function from webservice.php and check order, line item array, and based on this we set the status using getVendorgetInvoice and return array response.
3. VendorWebService.php
generatVendorOrder -
- In this function, we generate XML request for 3rd party suppliers.
Note - This file depends on supplier request format, parameters and its structure.
createVendorOrder -
- Based on the array received, we call generatVendorOrder which is defined in the same file.
- Then we post this request using the curl method, defined in this file itself and return error/status.
GetVendorOrderstatus -
- In this function we check connection based on order URL and curl post using get API and return response.
GetcheckAuthentication -
- In this function we check connection based on the supplier connection URL and curl post using get API and return response.
b) Chk Status w/ (Vendor name)
You can check the status of the order by clicking on the Check Status button.
Below are the related files:
4. Ordershipment.php
- In this file, based on the request parameters and POnumber, we check POStatus using the getPOStatus function which is defined in VendorMiddleware.php and return JSON response.
5. Testsupplierconnectorvendor.php
- In this file, we check authentication using checkAuthentication which is defined in VendorMiddleware.php and return status.
Note - In the above files, we also write logs using monolog
>>>Sample Code
<?php
/***************************************************************************
* AGILIRON Inc. (TM) - Accelerate Commerce. On Demand (SM)
* Support: [email protected], 1-800-853-8712
*
* Copyright (c) 2021 AGILIRON Inc. | All Rights Reserved.
*
* Unauthorized copying of this file, via any medium is strictly prohibited.
* Proprietary and Confidential.
*
****************************************************************************/
/*
* call by following
* https://company.agiliron.net/agiliron/index.php?action=createVendorSalesOrder&module=Orders&record=xxxx
* xxxx is the orderno(PO)
*
* This file will send create Order request to vendor supplier.
**/
require_once ('supplierconnectors/vendor/VendorMiddleware.php');
$userid = $_REQUEST['parameter1'];
$password = $_REQUEST['parameter2'];
$account = $_REQUEST['parameter3'];
$xml_string = $_REQUEST['podata'];
$xml = simplexml_load_string($xml_string);
$json = json_encode($xml);
$POdata = json_decode($json,TRUE);
$response = 'Supplier Integration Missing with Vendor';
if($userid != '' && $password != ''){
// class to initialize with param userid and password.
$VendorMiddleware = new VendorMiddleware($userid, $password);
// Function createVendorOrder() for send request to vendor for create order
$responseArr = $VendorMiddleware->createVendorOrder($POdata);
if($responseArr['status'] != 200){
$response = 'Error : '.$responseArr['error'];
}else{
if(isset($responseArr['OrderAddResponse']['order'])){
$status = $responseArr['OrderAddResponse']['order']['acceptreject'];
$itemstatus ='';
if(isset($responseArr['OrderAddResponse']['order']['item']['modelnumber'])){
$itmes = $responseArr['OrderAddResponse']['order']['item'];
$itemstatus .= '<br><br>Model Number :'.$itmes['modelnumber'];
$itemstatus .= '<br> Item Status :'.$itmes['acceptreject'];
if(isset($itmes['issue'])){
$itemstatus .= '<br> Error :'.$itmes['issue'];
}
}else{
foreach ($responseArr['OrderAddResponse']['order']['item'] as $itmes){
$itemstatus .= '<br><br>Model Number :'.$itmes['modelnumber'];
$itemstatus .= '<br> Item Status :'.$itmes['acceptreject'];
if(isset($itmes['issue'])){
$itemstatus .= '<br> Error :'.$itmes['issue'];
}
}
}
$response = '<b>Order Status :</b> '.$status;
$response .= '<br><br><b> Item Details : </b>'.$itemstatus;
}else{
$status = $responseArr['OrderAddResponse']['@attributes']['statusMessage'];
$response = '<b>Order response :</b> '.$status;
}
}
}
echo $response;
?>
<?php
/***************************************************************************
* AGILIRON Inc. (TM) - Accelerate Commerce. On Demand (SM)
* Support: [email protected], 1-800-853-8712
*
* Copyright (c) 2021 AGILIRON Inc. | All Rights Reserved.
*
* Unauthorized copying of this file, via any medium is strictly prohibited.
* Proprietary and Confidential.
*
****************************************************************************/
class VendorMiddleware
{
public $userid = "";
public $password = "";
public $vendor;
/*
* Function to initialize the required data for authentication with the supplier.
* @param $userid, $password
*/
public function __construct($userid, $password)
{
$this->vendor = new VendorWebService($userid, $password);
}
/*
* Function createVendorOrder() to create order in supplier vendor
* @param $PODATA
* @return response from supplier
*/
public function createVendorOrder($PODATA)
{
$response = $this->vendor->createVendorOrder($PODATA);
return $response;
}
/*
* Function getPOStatus() to get an order from DB for supplier and checked status on supplier vendor and update the order in DB.
*/
public function getPOStatus($ponumber)
{
$returnArr = array();
$response = $this->vendor->getVendorOrderstatus($ponumber);
if (isset($response['OrderStatusResponse']) && $response['OrderStatusResponse']['@attributes']['statusCode'] == 0) {
$returnArr['status'] = true;
$orderdetails = $response['OrderStatusResponse']['order'];
$returnArr['ponumber'] = $orderdetails['ponumber'];
$returnArr['starkorderid'] = $orderdetails['starkorderid'];
$returnArr['orderstatus'] = $orderdetails['orderstatus'];
if($orderdetails['orderstatus'] =='does not exist'){
$returnArr['orderstatus'] = '';
$returnArr['status'] = false;
$returnArr['Message'] = 'does not exist';
}
$orderstatusarr = $orderdetails['invoice'];
if(!isset($orderstatusarr[0])){
$orderstatusarr[0] = $orderstatusarr;
}
$invoiceArr = array();
if(isset($orderstatusarr[0])){
foreach ($orderstatusarr as $inv => $invoicedetail) {
$invoiceArr[] = $invoicedetail['invoicenumber'];
if(isset($orderdetails['invoice']['item'][0])){
$i = 1;
foreach ($orderdetails['invoice']['item'] as $key => $lineItem) {
if(is_array($lineItem['trackingnumber'])){
$returnArr['orderitem'][$inv.$key]['shiptrackno'] = $lineItem['trackingnumber'][0];
}else{
$returnArr['orderitem'][$inv.$key]['shiptrackno'] = $lineItem['trackingnumber'];
}
if(is_array($lineItem['shipdate'])){
$returnArr['orderitem'][$inv.$key]['ShipDate'] = $lineItem['shipdate'][0];
}else{
$returnArr['orderitem'][$inv.$key]['ShipDate'] = $lineItem['shipdate'];
}
$returnArr['orderitem'][$inv.$key]['itemid'] = $lineItem['modelnumber'];
$returnArr['orderitem'][$inv.$key]['QuantityOrdered'] = $lineItem['qty'];
$returnArr['orderitem'][$inv.$key]['QuantityShipped'] = $lineItem['qty'];
$returnArr['orderitem'][$inv.$key]['OrderLineNumber'] = $i++;
}
}else{
if(is_array($invoicedetail['item']['trackingnumber'])){
$returnArr['orderitem'][$inv]['shiptrackno'] = $invoicedetail['item']['trackingnumber'][0];
}else{
$returnArr['orderitem'][$inv]['shiptrackno'] = $invoicedetail['item']['trackingnumber'];
}
if(is_array($invoicedetail['item']['shipdate'])){
$returnArr['orderitem'][$inv]['ShipDate'] = $invoicedetail['item']['shipdate'][0];
}else{
$returnArr['orderitem'][$inv]['ShipDate'] = $invoicedetail['item']['shipdate'];
}
$returnArr['orderitem'][$inv]['itemid'] = $invoicedetail['item']['modelnumber'];
$returnArr['orderitem'][$inv]['QuantityOrdered'] = $invoicedetail['item']['qty'];
$returnArr['orderitem'][$inv]['QuantityShipped'] = $invoicedetail['item']['qty'];
$returnArr['orderitem'][$inv]['OrderLineNumber'] = 1;
}
}
$returnArr['invoicenumber'] = implode(",", $invoiceArr);
}
}else{
$errormsg = $response['OrderStatusResponse']['@attributes']['statusMessage'];
if(isset($response['error']) && $response['error'] != ''){
$errormsg = $response['error'];
}
$returnArr['status'] = false;
$returnArr['Message'] = $errormsg;
}
return $returnArr;
}
/*
* Function checkAuthentication() to check supplier Authentication.
*/
public function checkAuthentication(){
$authReturn = $this->vendor->getcheckAuthentication();
return $authReturn;
}
}
?>
<?php
/***************************************************************************
* AGILIRON Inc. (TM) - Accelerate Commerce. On Demand (SM)
* Support: [email protected], 1-800-853-8712
*
* Copyright (c) 2021 AGILIRON Inc. | All Rights Reserved.
*
* Unauthorized copying of this file, via any medium is strictly prohibited.
* Proprietary and Confidential.
*
****************************************************************************/
/**
* Class WebService
* This class will a handler that will be used to create order and get order status from vendor Services
*/
class VendorWebService {
public $userid = "";
public $password = "";
public $orderurl = "";
public $itemsurl = "";
/*
* Function to initialize the required data for authentication with the supplier.
* @param $userid, $password
*/
function __construct($userid,$password){
$this->userid = $userid;
$this->password = $password;
// This is API url for vendor
$this->orderurl = 'http://api.vendorpremium.com/api/Service1.svc/orders';
$this->itemsurl = 'http://api.vendorpremium.com/api/Service1.svc/orderstatus';
}
/**
* Function generatVendorOrderXML() generate Order XML from Order Array
* @param $orderArr
* @return orderXML
*/
public function generatVendorOrderXML($orderArr) {
try{
$startorderitem = '';
foreach ($orderArr['OrderDetails'] as $items){
if(($items[0])){
foreach ($items as $item){
$startorderitem .= "<item>
<modelnumber>".$item['vendor_part_no']."</modelnumber>
<quantity>".$item['products_quantity']."</quantity>
<unitprice>".$item['products_price']."</unitprice>
<description>".cleanInput($item['detail_notes'])."</description>
</item>";
}
}else{
$startorderitem .= "<item>
<modelnumber>".$items['vendor_part_no']."</modelnumber>
<quantity>".$items['products_quantity']."</quantity>
<unitprice>".$items['products_price']."</unitprice>
<description>".cleanInput($items['detail_notes'])."</description>
</item>";
}
}
$XPost = "<Vendor>
<orders>
<access>
<userid>".$this->userid."</userid>
<password>".$this->password."</password>
</access>
<order>
<ponumber>test_".$orderArr['orderno']."</ponumber>
<comments>".$orderArr['description']."</comments>
<shipto>
<name>Test</name>
<address1>".$orderArr['delivery_street_address']."</address1>
<city>".$orderArr['delivery_city']."</city>
<state>".$orderArr['delivery_state']."</state>
<zip>".$orderArr['delivery_postcode']."</zip>
</shipto>
$startorderitem
</order>
</orders>
</Vendor>";
return $XPost;
} catch (Exception $ex) {
$error = "Error IN function generatVendorOrderXML ".$ex->errorMessage();
$this->logToFileVendor($error);
}
}
/**
* Function generatVendorgetstatusXML() for generat XML for Order status.
* @param $orderNumberArr
* @return orderXML
*/
public function generatVendorgetstatusXML($orderNumberArr) {
try{
$orderXML = '';
foreach ($orderNumberArr as $orderNumber){
$orderXML .= "<order>
<ponumber>".$orderNumber."</ponumber>
</order>";
}
$XPost = "<Vendor>
<orders>
<access>
<userid>".$this->userid."</userid>
<password>".$this->password."</password>
</access>
$orderXML
</orders>
</Vendor>";
return $XPost;
} catch (Exception $ex) {
$error = "Error IN function generatVendorgetstatusXML ".$ex->errorMessage();
$this->logToFileVendor($error);
}
}
/**
* Function createVendorOrder() to call request for vendor supplier and return response from the vendor.
* @param $orderArr
* @return mixed
*/
public function createVendorOrder($orderArr) {
try{
$OXML = $this->generatVendorOrderXML($orderArr);
$return = $this->_post($this->orderurl,$OXML);
return $return;
} catch (Exception $ex) {
$error = "Error IN function createVendorOrder ".$ex->errorMessage();
$this->logToFileVendor($error);
}
}
/**
* Function getVendorOrderstatus() to get Order status from vendor supplier.
* @param $orderNumberArr
* @return mixed
*/
public function getVendorOrderstatus($orderNumberArr) {
try {
$OXML= $this->generatVendorgetstatusXML($orderNumberArr);
$return = $this->_post($this->orderurl, $OXML);
return $return;
} catch (Exception $ex) {
$error = "Error IN function getVendorOrderstatus ".$ex->errorMessage();
$this->logToFileVendor($error);
}
}
/**
* Function checkAuthentication() get check Authentication with vendor supplier.
* @return mixed
*/
public function getcheckAuthentication() {
try {
$XPost = "<Vendor>
<orders>
<access>
<userid>".$this->userid."</userid>
<password>".$this->password."</password>
</access>
</orders>
</Vendor>";
$return = $this->_post($this->orderurl,$XPost);
return $return;
} catch (Exception $ex) {
$error = "Error IN function getcheckAuthentication ".$ex->errorMessage();
$this->logToFileVendor($error);
}
}
/**
* Function getStockItem() we not use this for now.
* @param $orderXML
* @return mixed
*/
public function getVendorItem($orderXML) {
$return = $this->_post($this->itemsurl,$orderXML);
return $return;
}
/**
* Function _get() for API call with Method GET
* @param $uri
* @param $XML
* @return mixed
*/
public function _get($uri,$XML) {
return $this->_call("GET",$uri,$XML);
}
/**
* Function _post() for API call with Method POST
* @param $uri
* @param $XML
* @return mixed
*/
public function _post($uri,$XML) {
return $this->_call("POST",$uri,$XML);
}
/**
* Function _call() make an API calls with XML to vendor supplier.
* @param $type
* @param $uri
* @param $XML
* @return mixed
*/
public function _call($type,$uri,$XML) {
try {
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => $uri,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => $XML,
CURLOPT_HTTPHEADER => array(
"cache-control: no-cache",
"content-type: text/xml",
),
));
$response = curl_exec($curl);
$info = curl_getinfo($curl);
$error = curl_error ( $curl );
$xml = simplexml_load_string($response);
$json = json_encode($xml);
$responsearray = json_decode($json, TRUE);
$responsearray['status'] = $info['http_code'];
$responsearray['error'] = $error;
return $responsearray;
} catch (Exception $ex) {
$error = "Error IN function _call ".$ex->errorMessage();
$this->logToFileVendor($error);
return $error;
}
}
/**
* Function logToFileVendor() to wirte error log.
* @param $textString
*/
function logToFileVendor($textString) {
try {
$logFile = fopen(dirname(__FILE__) . "/vendorlog.log", 'a');
fwrite($logFile, serialize($textString));
fwrite($logFile, "\n");
fclose($logFile);
} catch (Exception $ex) {
echo "Error on LogToFile.php";
}
}
}
?>
<?php
/***************************************************************************
* AGILIRON Inc. (TM) - Accelerate Commerce. On Demand (SM)
* Support: [email protected], 1-800-853-8712
*
* Copyright (c) 2021 AGILIRON Inc. | All Rights Reserved.
*
* Unauthorized copying of this file, via any medium is strictly prohibited.
* Proprietary and Confidential.
*
****************************************************************************/
/*
* This file for update order status from vendor
* @request parameter can used for get order status from vendor username,password
**/
// include this file for get use class / method.
require_once ('supplierconnectors/vendor/VendorMiddleware.php');
$userid = $_REQUEST['parameter1'];
$password = $_REQUEST['parameter2'];
$account = $_REQUEST['parameter3'];
$ponumber = $_REQUEST['ponumber'];
if($userid != '' && $password != '') {
// class to initialize with param userid and password.
$VendorMiddleware = new VendorMiddleware($userid, $password);
// Function getPOStatus() for get order status from supplier.
$response = $VendorMiddleware->getPOStatus($ponumber);
if($response != ''){
echo $response;
}else{
echo "process completed";
}
}
?>
<?php
/***************************************************************************
* AGILIRON Inc. (TM) - Accelerate Commerce. On Demand (SM)
* Support: [email protected], 1-800-853-8712
*
* Copyright (c) 2021 AGILIRON Inc. | All Rights Reserved.
*
* Unauthorized copying of this file, via any medium is strictly prohibited.
* Proprietary and Confidential.
*
****************************************************************************/
/**
* This file for test supplier connection.
* @request parameter can used for check authentication from vendor username,password
**/
// include this file for get use class / method.
require_once('supplierconnectors/vendor/VendorMiddleware.php');
$userid = $_REQUEST['username'];
$password = $_REQUEST['password'];
$responseArr = array();
// class to initialize with param userid and password.
$VendorMiddleware = new VendorMiddleware($userid, $password);
// Function checkAuthentication() for get authentication from supplier.
$returnAuth = $VendorMiddleware->checkAuthentication();
if(isset($returnAuth['OrderAddResponse']['@attributes']['statusCode']) && $returnAuth['OrderAddResponse']['@attributes']['statusCode'] == 0){
$statusMessage = 'Test connection successful';
$status = true;
}else{
$statusMessage = 'Test connection failed';
$status = false;
}
$responseArr['status'] = $status;
$responseArr['msg'] = $statusMessage;
echo json_encode($responseArr);
exit;
?>
Updated 5 months ago